· tutorials · 3 min read
Simplifying Login Automation with Puppeteer
Welcome back to our series on writing automation scripts! In this post, we're diving into a straightforward approach to automate login processes using Puppeteer—a powerful tool for browser automation. We'll walk through handling username and password fields, as well as leveraging cookies for efficient logins.
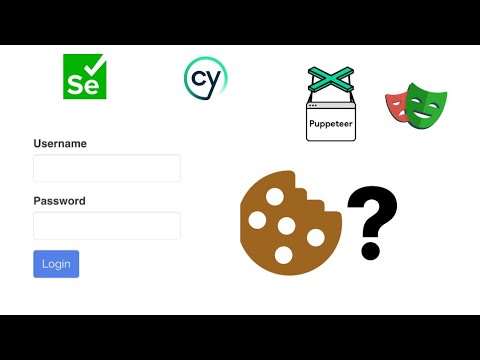
This blog was generated from a tutorial video you can watch here
Setting Up for Login Automation
Let’s start with a sample website. We’re going to show how to log in using Puppeteer by automating the entry of credentials. Imagine a simple login screen where you input a username and password. For our demo, we’ll use the credentials:
- Username: automize
- Password: 123456
When you log in successfully, the interface will confirm your logged-in status by replacing the login button with a logout button. Simple enough, right?
Using Puppeteer for Automation
To implement this in Puppeteer, we’ll follow a few key steps. Here’s a high-level overview of the process:
- Initiate Puppeteer: First, we navigate to the login URL.
- Interact with Fields: We’ll locate the username and password fields and input our credentials.
- Submit the Form: Finally, we’ll click the login button to authenticate.
Here’s how that might look in code:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('your-login-url-here');
// Type in the username
await page.type('input[name="username"]', 'automize');
// Type in the password
await page.type('input[name="password"]', '123456');
// Click the login button
await page.click('button[type="submit"]');
// Wait for a confirmation that we're logged in
await page.waitForSelector('selector-for-logged-in-state');
console.log('Logged in!');
await browser.close();
})();
In this script, we send the credentials directly to the input fields. Note that for security reasons, you generally wouldn’t want to hard-code passwords in production scripts.
Bypassing Login with Cookies
As we mentioned, login scenarios can get complicated, especially when they involve additional authentication methods like email verification or SMS codes. So, how does the web application keep track of whether we are logged in? It uses cookies!
Using Cookies
When you log in, the server issues a session token stored as a cookie in your browser. This cookie is critical as it contains your authentication details. Instead of logging in repeatedly, you can use Puppeteer to send this existing session token to authenticate automatically.
- Capture the Cookie: Monitor the Network tab in your browser while logging in to capture the session cookie.
- Implement the Cookie in Puppeteer: Modify your Puppeteer script to include this cookie, avoiding the need for manual login each time.
Here’s how you can integrate the cookie into your Puppeteer script:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
// Set the cookie before navigating
const cookie = {
name: 'session',
value: 'your-session-token',
domain: 'your-domain.com',
};
await page.setCookie(cookie);
await page.goto('your-site-url');
// Now you should be logged in
console.log('Logged in with cookie!');
await browser.close();
})();
Important Considerations
- Cookie Expiration: Keep in mind that session tokens expire, often within 30 minutes to an hour. You’ll need to update your cookies regularly to maintain login access.
- Security Practices: Avoid using sensitive information directly in scripts. Always follow best security practices to protect user data.
Conclusion
Automating login processes with Puppeteer can greatly streamline repetitive tasks, whether you’re testing web applications or scraping data. By leveraging cookies, you can enhance efficiency and avoid unnecessary hassle with login forms.
If you have any questions or would like to see more about Puppeteer or automation scripts, feel free to leave a comment. Happy