· tutorials · 3 min read
Streamlining Your Web Scraping Process with JavaScript
Welcome back to our series on writing automation scripts! Today, we’re enhancing our previous automation script by employing smarter methods for web scraping. In this post, we’ll dive into a practical example of using JavaScript to efficiently extract data from a webpage.
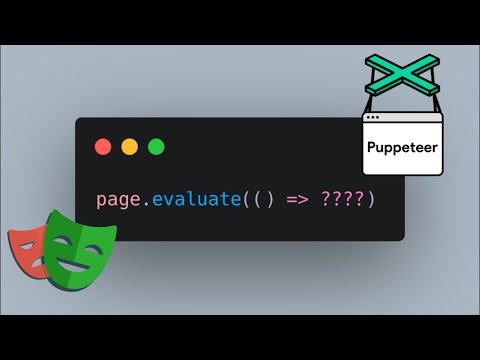
This blog was generated from a tutorial video you can watch here
The Importance of Preliminary Investigation
Before jumping into writing your automation scripts, it’s crucial to conduct some preliminary investigation. This can save you significant time and effort down the line. One of the first steps I like to take is to check whether the data we need is being pulled from the server or if it’s simply rendered on the page.
Using Developer Tools
To determine how the data is served, you can utilize your browser’s Developer Tools. Open the Network tab, refresh the page, and observe the requests made. In many scenarios, the data will not be visible in the XHR requests, leading us to conclude that the information is being rendered directly in the HTML source.
You can also right-click and select “View Page Source” to examine the HTML. Often, you’ll find your desired data located right in the source code, which can simplify the scraping process tremendously.
Accessing Data with JavaScript
After determining that the data exists in the page source, you can easily access this information directly via the console. For instance, typing in window.data
can provide you insights into the data structure that is rendered on your screen. This method is not only more straightforward but also more robust, as it often gives you the exact data format you need.
In our case, we found the data was presented in a structured format, which included properties like names, links, and tags. This allowed us to streamline our scraping process significantly.
Simplifying the Scraping Code
With the raw data readily available in window.data
, we can simplify our scraping code. Instead of executing a lengthy scraping routine, we can directly reference this data.
Utilizing JavaScript, we can evaluate code within the browser’s context to extract the data efficiently. By copying the object from the console, you can work with this JSON data, or you can use a function to return and log it.
Here’s a basic overview of what our code looks like:
let data = window.data;
console.log(data);
This line retrieves our structured data, making it ready for further processing.
Looping Through the Data
Now that we have access to the data, we can use simple loops to iterate through it. Here’s an example:
for (let i = 0; i < data.length; i++) {
const quote = data[i];
const tags = quote.tags.join(', ');
const text = quote.text;
const author = quote.author.name;
// Output or save your data
}
This loop extracts tags, text, and the author for each entry in our dataset, providing a clean output of the necessary information.
Conclusion
As we’ve demonstrated, there are multiple ways to scrape data from a website, and you don’t always have to rely on traditional scraping methods. By investigating how data is rendered on the webpage and utilizing browser capabilities, you can streamline your scraping efforts significantly.
Thank you for joining us today! If you have any video ideas or feature requests for future tutorials on Automize, please share them in the comments. Stay tuned for the next installment, where we’ll explore more innovative approaches to web sc