· tutorials · 2 min read
Automating Printer Searches on Best Buy with Playwright
In today’s post, we’ll dive into writing automation scripts using Playwright, focusing on how to efficiently search for printers on Best Buy's website. Playwright, developed by Microsoft, is a powerful tool for automating web interactions. We’ll be using JavaScript for this task, so let’s get started!
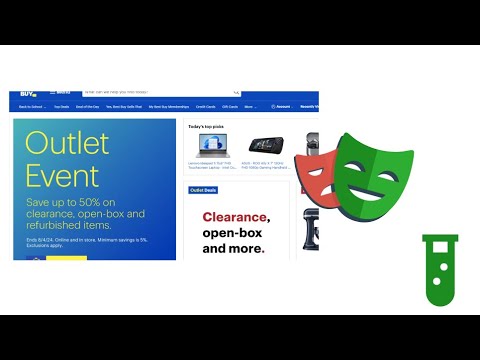
This blog was generated from a tutorial video you can watch here
Setting Up the Script
Begin by creating a simple script that navigates to the Best Buy website. Once your code is set up, you can execute it to confirm it opens the site in a browser window.
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://www.bestbuy.com/');
})();
Searching for Printers
Now that we’re on Best Buy’s homepage, the next step is to search for printers. We’ll type ‘printer’ into the search bar and click on the first relevant result. This process involves using automated input simulation to interact with the webpage.
await page.fill('input[type="text"]', 'printer');
await page.click('text=Wireless Printers');
Extracting Results
Once we click on the desired search result, the next objective is to extract the list of printers displayed on the page. To do this, we’ll identify the relevant elements and loop through them to retrieve the details, such as price and title.
First, we can select all the printer items listed:
let items = await page.$$('div[data-item-id]');
Then, we can iterate through these items:
for (let item of items) {
const title = await item.$eval('h4', el => el.innerText);
const price = await item.$eval('.price', el => el.innerText);
console.log(`Title: ${title}, Price: ${price}`);
}
Enhancing the Script
To ensure robustness, we need to account for changes in product attributes. Instead of hardcoding specific attributes such as ‘Wireless’, which may change as technology evolves, we can utilize more stable selectors. For instance, using data-test-id
will yield more adaptable results as the product categories update over time.
await page.click('[data-test-id="item-0"]'); // Using a data attribute for selection
Finalizing the Script
After assembling the key elements and iterating through them, ensure you implement necessary waits to allow page elements to load before extraction. This guarantees that you retrieve the latest data without running into timing issues.
await page.waitForSelector('.sku-item');
Conclusion
With this foundational knowledge, you’re now equipped to enhance your web automation skills using Playwright. Not only can you search for printers effectively now, but this method can also be adapted for various products across multiple e-commerce platforms. In our next session, we’ll refine and add tests to improve our script’s reliability.
Stay tuned for more automation tips and t