· tutorials · 4 min read
Automating the Web A Puppeteer Primer and the Power of Streamlined Scripting
Explore Puppeteer basics in JavaScript, then discover how Automize enhances web automation with AI, seamless interfaces, and versatile compatibility.
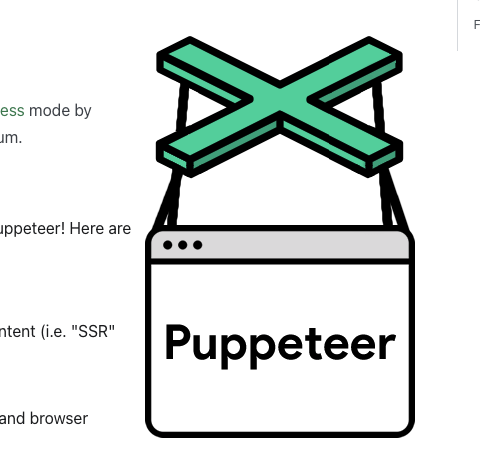
An Introduction to Puppeteer: Automating Web Browsing with JavaScript
Web automation has become an integral part of modern web development and testing workflows. One powerful tool that developers often turn to for automating browser tasks is Puppeteer. Puppeteer is a Node library developed by the Chrome team at Google. It provides a high-level API to control headless browsers or full browsers over the DevTools Protocol.
Understanding Puppeteer
Installing Puppeteer
Before diving into examples, it’s important to install Puppeteer. You can do this by using npm:
npm install puppeteer
Once installed, you can require Puppeteer in your JavaScript file:
const puppeteer = require('puppeteer');
Launching a Browser Instance
Puppeteer allows you to launch a new browser instance. Here’s a simple example:
const browser = await puppeteer.launch();
const page = await browser.newPage();
The launch
method launches a new browser instance, and newPage
creates a new page within that instance.
Navigating to a Website
Let’s navigate to a website:
await page.goto('https://example.com');
Clicking Buttons
To interact with elements on a page, Puppeteer provides the click
method. Let’s click a button:
await page.click('button#submit');
Entering Text
Entering text into input fields is a common automation task. Puppeteer simplifies this process:
await page.type('input#username', 'your_username');
await page.type('input#password', 'your_password');
Assertions
Puppeteer enables you to perform assertions on the content of a page. For example, checking if an element with a specific selector exists:
const elementExists = await page.$('h1#pageTitle');
console.log('Element exists:', !!elementExists);
Working with Iframes
Handling iframes in Puppeteer is straightforward. You can switch to an iframe using the frame
method:
const iframeHandle = await page.$('iframe#iframeId');
const iframe = await iframeHandle.contentFrame();
Putting It All Together
Now, let’s create a complete example that navigates to a website, clicks a button, enters text, performs assertions, and interacts with an iframe:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
// Navigate to a website
await page.goto('https://example.com');
// Click a button
await page.click('button#submit');
// Enter text
await page.type('input#username', 'your_username');
await page.type('input#password', 'your_password');
// Perform assertions
const pageTitle = await page.title();
console.log('Page title:', pageTitle);
// Work with iframes
const iframeHandle = await page.$('iframe#iframeId');
const iframe = await iframeHandle.contentFrame();
// Close the browser
await browser.close();
})();
Introduction to Automize: Simplifying Web Automation
While Puppeteer is a powerful tool for web automation in JavaScript, there are cases where developers may seek additional features or a more streamlined workflow. This is where Automize comes into play. Automize is a Chrome extension designed to automate the process of writing scripts to interact with websites.
Integrating with Puppeteer
Automize seamlessly integrates with Puppeteer, providing a user-friendly interface within Chrome DevTools. This makes it easier to write automation scripts directly in the browser, enhancing the development process.
AI-Powered Automation
Automize leverages AI technology for auto element selection, making it easier to select elements intelligently without manual intervention. This can significantly speed up the scripting process and reduce the chances of errors.
Quick Verification and Excellent Support
Automize provides features like quick verification of selectors and excellent customer support, ensuring a smooth and efficient web automation experience. Developers can quickly identify and rectify issues, thanks to Automize’s responsive support options.
Comprehensive Language and Framework Support
Automize is not limited to Puppeteer; it works seamlessly with Playwright, Cypress, Selenium, XPath, CSS, and more. This broad compatibility ensures that developers can choose the tools and frameworks that best suit their needs.
Network Events and Mocking Capabilities
With Automize, developers can easily mock, assert, test, and write code for network events directly within the tool. This is a valuable feature for comprehensive testing and debugging scenarios.
Effortless Selection and Code Conversion
Automize simplifies the scripting process with an intuitive interface for effortless element selection. Additionally, it facilitates the conversion of actions into clean, reusable code snippets for Puppeteer, Cypress, and Playwright.
Conclusion: Elevating Your Web Development Journey
In conclusion, Puppeteer is a robust and versatile tool for automating browser tasks using JavaScript. However, for developers looking to streamline their workflow, enhance AI-driven element selection, and benefit from additional features, Automize serves as a valuable extension.
Whether you are automating web interactions, conducting robust testing, or working with iframes, Automize provides a user-friendly interface and advanced capabilities. Its compatibility with popular frameworks and languages, coupled with AI integration, makes it a compelling choice for developers seeking a comprehensive web automation solution.
To empower your web development journey with seamless automation and effortless scripting, consider exploring Automize as a complementary tool to Puppeteer. Together, these tools can significantly enhance your ability to write, test, and maintain automation scripts, ultimately contributing to a more efficient and productive development process.