· tutorials · 4 min read
Introduction to Playwright
Mastering Web Automation with Playwright A Comprehensive Guide in JavaScript
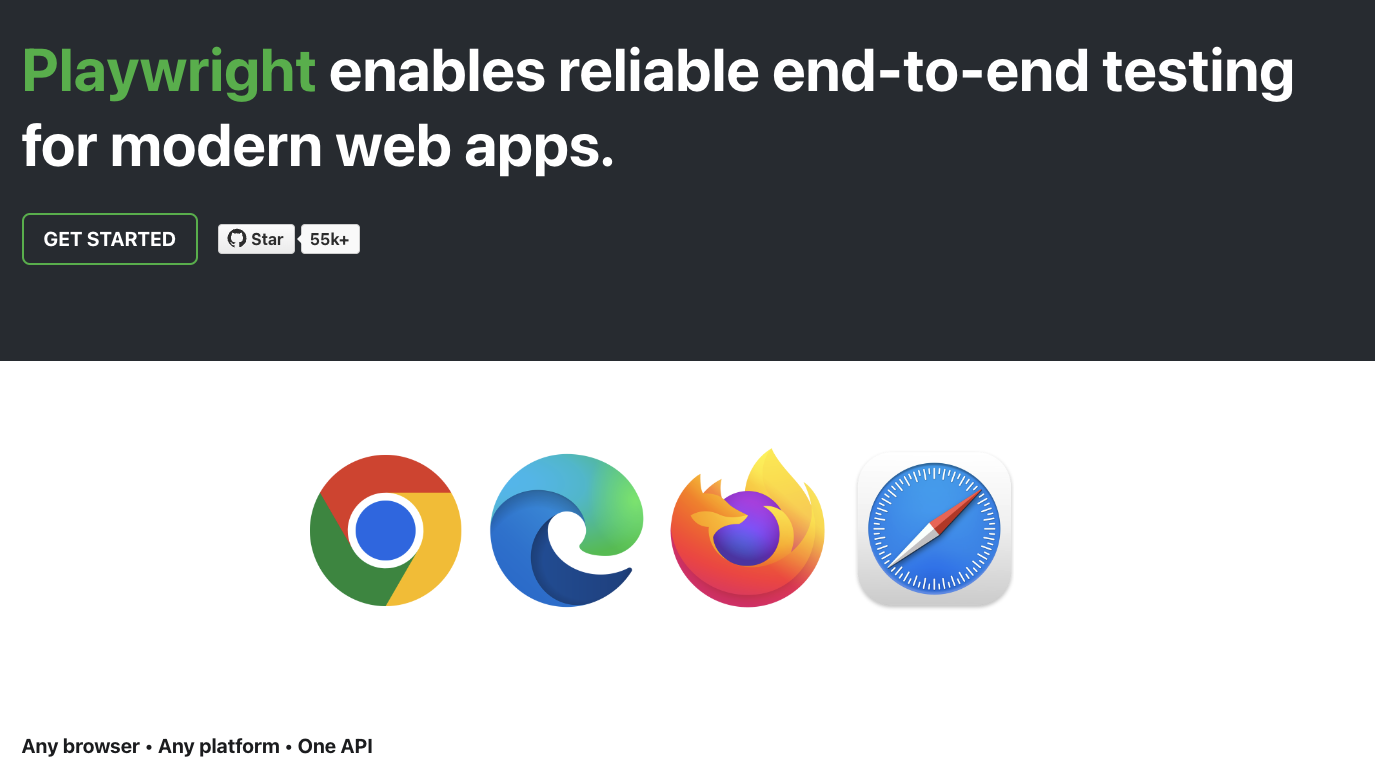
In the ever-evolving landscape of web development, automation plays a pivotal role in streamlining workflows, enhancing efficiency, and ensuring the robustness of web applications. Among the array of tools available for web automation, Playwright has emerged as a powerful and versatile solution, particularly in the realm of browser automation. In this comprehensive guide, we’ll delve into the fundamentals of Playwright, exploring its capabilities through practical examples in JavaScript.
Understanding Playwright
Playwright is an open-source automation tool developed by Microsoft, designed to automate the interaction of web browsers. What sets Playwright apart is its cross-browser compatibility, supporting major browsers like Chrome, Firefox, and Safari. It provides a unified API to automate web pages across different browsers, making it a go-to choice for developers seeking a versatile and comprehensive automation solution.
Getting Started with Playwright
Before we dive into the intricacies of Playwright, let’s ensure you have it set up in your development environment. Follow these simple steps to get started:
Installation: Install Playwright using npm:
npm install playwright
Launch a Browser Instance: Use Playwright to launch a browser instance (e.g., Chromium):
const { chromium } = require('playwright'); (async () => { const browser = await chromium.launch(); const context = await browser.newContext(); const page = await context.newPage(); // Your automation code goes here await browser.close(); })();
With Playwright set up, let’s explore some common automation scenarios.
Automating Interactions with Playwright in JavaScript
1. Clicking Buttons
Clicking buttons is a fundamental aspect of web automation. Here’s how you can achieve this with Playwright:
// Clicking a button with Playwright
await page.click('button#submitBtn');
2. Entering Text
Input fields are ubiquitous on the web, and automating text entry is a common requirement:
// Entering text into an input field with Playwright
await page.type('input#username', 'JohnDoe');
3. Assertions
Assertions are crucial for validating the behavior of web elements. Playwright provides robust methods for assertions:
// Asserting the presence of an element
const elementExists = await page.waitForSelector('div#result');
expect(elementExists).toBeTruthy();
4. Working with Iframes
Playwright simplifies the process of interacting with iframes, allowing you to seamlessly switch contexts:
// Switching to an iframe with Playwright
const iframeHandle = await page.waitForSelector('iframe#iframe1');
const iframe = await iframeHandle.contentFrame();
// Now you can interact with elements inside the iframe
await iframe.click('button#iframeBtn');
By mastering these fundamental interactions, you gain a solid foundation for more complex automation scenarios.
Why Playwright?
Playwright’s popularity can be attributed to several key features:
Cross-Browser Compatibility: Playwright supports multiple browsers, allowing developers to write consistent automation scripts regardless of the browser being used.
Rich API: Playwright provides a comprehensive API for browser automation, covering a wide range of actions and scenarios.
Performance: Playwright is designed for speed, making it efficient for both simple and complex automation tasks.
Community Support: Being an open-source project, Playwright benefits from a vibrant community that actively contributes to its development and provides support.
Introducing Automize: Elevating Your Web Automation Experience
As you explore the capabilities of Playwright, you might find yourself seeking additional tools to enhance your automation workflow. This is where Automize comes into play. Automize is a cutting-edge Chrome extension that takes web automation to the next level.
Automize and Playwright Integration
Automize seamlessly integrates with Playwright, offering a streamlined interface for script creation. With features like auto element selection and AI integration, Automize complements Playwright’s capabilities, making the process of writing automation scripts even more intuitive.
How Automize Enhances Playwright Automation:
Effortless Element Selection: Automize’s intuitive interface simplifies element selection, ensuring that even complex tasks are reduced to a few clicks.
AI Integration: Leveraging sophisticated AI technology, Automize optimizes selector choices, instilling confidence in the reliability of generated selectors.
Network Event Support: Automize extends Playwright’s capabilities with advanced network event features, facilitating comprehensive testing scenarios.
Iframe Support: Seamlessly work with iframes using Automize and export your scripts to Playwright effortlessly.
Code Conversion: Transform your actions into clean, reusable code snippets for Puppeteer, Cypress, and Playwright with Automize.
Getting Started with Automize:
Installation: Simply install the Automize Chrome extension and start automating directly from Chrome DevTools.
Effortless Scripting: Automize operates within the Chrome browser, eliminating the need for additional setup steps. Write robust automation scripts quickly and without frustration.
Frequent Updates: Automize is committed to continuous improvement. Since its launch in July 2023, it has undergone 11 updates, ensuring users receive the latest features and enhancements.
Conclusion
In conclusion, Playwright stands as a robust choice for web automation in JavaScript, offering a powerful and versatile solution for developers. When coupled with Automize, the automation experience reaches new heights, combining the strengths of Playwright with an intuitive interface, AI integration, and advanced features. Whether you’re a seasoned developer or just getting started with web automation, the combination of Playwright and Automize provides a comprehensive toolkit to elevate your development journey. Embrace the power of automation, simplify your scripting process, and unlock new possibilities in web development. Get started with Playwright, and consider Automize for a seamless and enhanced automation experience.