· tutorials · 6 min read
Introduction to Cypress
Explore Cypress.js for efficient web testing. Learn button clicks, text entry, assertions, and iframe handling using JavaScript examples.
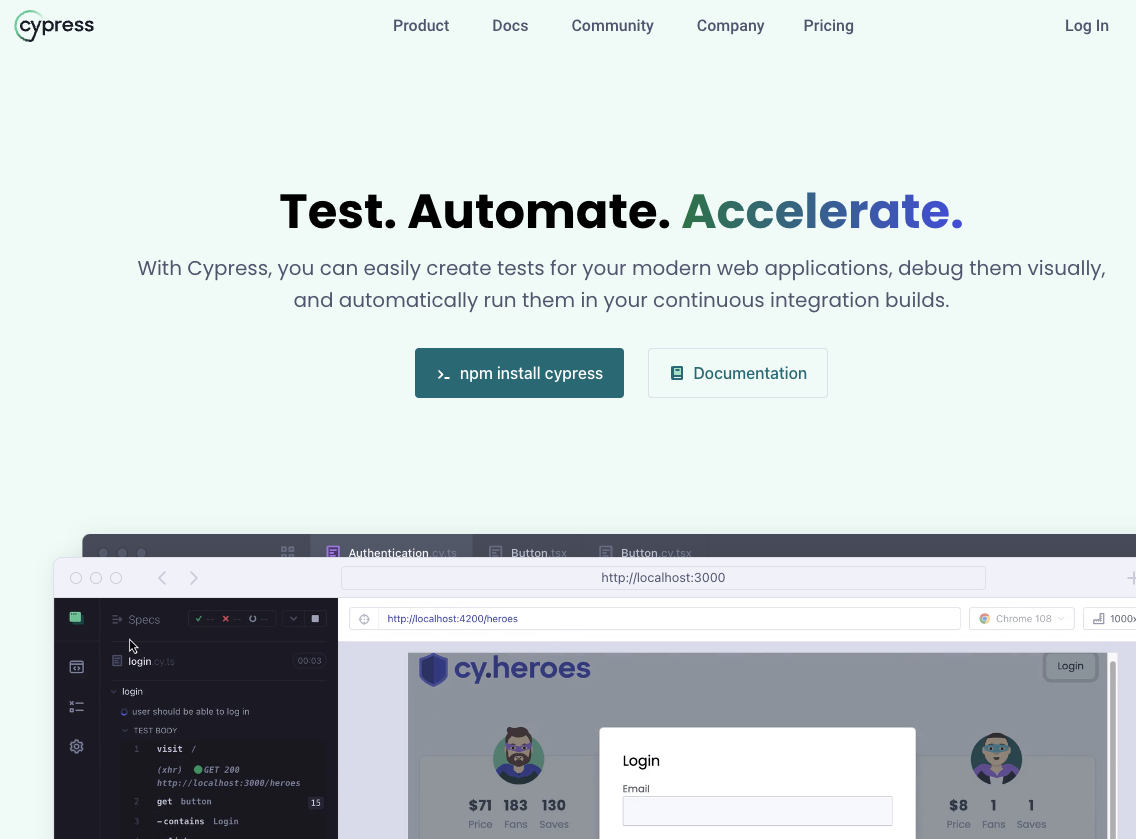
Cypress.js: A Comprehensive Guide to Automated Testing
Introduction
Cypress.js has emerged as a powerful and developer-friendly end-to-end testing framework for modern web applications. Its robust capabilities, intuitive syntax, and real-time feedback make it a preferred choice for many developers. In this comprehensive guide, we will delve into the key features of Cypress.js, covering everything from basic interactions like clicking buttons and entering text to more advanced scenarios involving assertions and handling iframes.
What is Cypress.js?
Cypress.js is an open-source JavaScript end-to-end testing framework that facilitates easy and effective testing of web applications. Unlike traditional testing tools, Cypress operates directly in the browser, providing fast, reliable, and consistent results. It is specifically designed to simplify the process of testing web applications, making it an excellent choice for developers who value efficiency and ease of use.
Getting Started with Cypress.js
Before we dive into the specifics of Cypress.js, let’s make sure you have it set up on your system. Follow these steps to install Cypress:
# Install Cypress as a dev dependency in your project
npm install --save-dev cypress
Once Cypress is installed, you can open the Cypress Test Runner with the following command:
npx cypress open
This will launch the Cypress Test Runner, allowing you to create and run tests interactively.
Basic Interactions
Clicking a Button
Clicking a button is one of the most common interactions in web applications. In Cypress, this can be achieved using the click()
command. Let’s consider a scenario where we have a button with the id “submitBtn,” and we want to click it:
// cypress/integration/button.spec.js
describe('Button Click Test', () => {
it('Clicks the submit button', () => {
// Visit the page with the button
cy.visit('https://example.com');
// Click the button with id "submitBtn"
cy.get('#submitBtn').click();
// Add assertions or further actions as needed
});
});
Entering Text
Typing into input fields is another fundamental interaction. Cypress provides the type()
command for this purpose. Suppose we have a login form with username and password fields:
// cypress/integration/form.spec.js
describe('Form Interaction Test', () => {
it('Types into the username and password fields', () => {
// Visit the page with the form
cy.visit('https://example.com/login');
// Type into the username field
cy.get('#username').type('john_doe');
// Type into the password field
cy.get('#password').type('securePassword123');
// Add assertions or further actions as needed
});
});
Assertions
Assertions are crucial for verifying that your application behaves as expected. Cypress makes it easy to perform assertions using its built-in commands. Let’s look at an example where we assert that clicking a button triggers a specific action:
// cypress/integration/assertions.spec.js
describe('Assertions Test', () => {
it('Verifies the action after clicking a button', () => {
// Visit the page with the button
cy.visit('https://example.com');
// Click the button with id "actionBtn"
cy.get('#actionBtn').click();
// Assert that a certain element is visible after the click
cy.get('#resultMessage').should('be.visible');
// Add more assertions as needed
});
});
Handling Iframes
Handling iframes in Cypress involves switching the context to the iframe before performing any interactions. Let’s consider a scenario where we have an iframe with a form inside it:
// cypress/integration/iframe.spec.js
describe('Iframe Interaction Test', () => {
it('Fills out a form inside an iframe', () => {
// Visit the page with the iframe
cy.visit('https://example.com/iframe-page');
// Switch to the iframe with id "iframeId"
cy.get('iframe#iframeId').iframe().within(() => {
// Now, we are inside the iframe context
// Type into the input field inside the iframe
cy.get('#iframeInput').type('Hello, Cypress!');
// Click the submit button inside the iframe
cy.get('#iframeSubmitBtn').click();
});
// Add assertions or further actions outside the iframe as needed
});
});
In this example, the cy.get('iframe#iframeId').iframe().within()
command is used to switch the context to the iframe. All subsequent commands will be executed within the iframe until the within
block ends.
Advanced Usage
Cypress.js offers advanced features that can enhance your testing workflow. Some notable features include:
Custom Commands
You can create custom commands to encapsulate repetitive actions or assertions. For instance, suppose you frequently need to log in during your tests. You can create a custom command to simplify this process:
// cypress/support/commands.js
Cypress.Commands.add('login', (username, password) => {
cy.visit('https://example.com/login');
cy.get('#username').type(username);
cy.get('#password').type(password);
cy.get('#loginBtn').click();
});
Now, you can use the cy.login()
command in your tests:
// cypress/integration/custom-command.spec.js
describe('Custom Command Test', () => {
it('Logs in using a custom command', () => {
cy.login('john_doe', 'securePassword123');
// Continue with assertions or further actions
});
});
Network Requests
Cypress allows you to intercept and modify network requests, enabling you to control the behavior of your application under different scenarios. For example, you can stub a network request to simulate various responses:
// cypress/integration/network-requests.spec.js
describe('Network Requests Test', () => {
it('Intercepts and modifies a network request', () => {
// Intercept a specific API endpoint
cy.intercept('GET', '/api/data', { fixture: 'sample-data.json' }).as('getData');
// Trigger the action that makes the network request
cy.get('#loadDataBtn').click();
// Wait for the intercepted request to complete
cy.wait('@getData');
// Add assertions based on the modified response
cy.get('#dataContainer').should('contain', 'Sample Data');
});
});
Best Practices
To make the most of Cypress.js, consider the following best practices:
Isolate Tests: Ensure that each test is independent and doesn’t rely on the state of other tests. This makes it easier to identify and fix issues.
Use Fixtures: Store test data in fixtures to keep test data separate from test code. This enhances maintainability and readability.
Avoid
cy.wait()
: Minimize the use ofcy.wait()
as much as possible. Cypress provides powerful commands likecy.get()
with built-in retry mechanisms, reducing the need for explicit waits.Organize Test Files: Organize your test files in a logical structure. For example, group tests related to user authentication in one folder and those related to form interactions in another.
Automize
Automize is a tool for web scraping that helps you automate repetitive tasks and ensure a positive scraping experience for both you and the website owners. If you don’t want to create all the selectors shown in this guide, you can use Automize’s AI tool to select them for you! Automize supports Playwright, Puppeteer, Cypress and more.
Conclusion
Cypress.js has revolutionized automated testing, offering developers an efficient and user-friendly framework. With its intuitive syntax and powerful features, it simplifies the testing process, from basic interactions like button clicks and text entry to advanced scenarios such as assertions and iframe handling. By incorporating best practices, custom commands, and network request control, developers can maximize the potential of Cypress.js, ensuring robust and reliable testing for modern web applications. Embrace the power of Cypress.js to elevate your testing workflow and build more resilient and high-quality web applications. Happy testing!