· tutorials · 2 min read
Streamlining Web Scraping with Automation Scripts
Welcome back to our series on writing automation scripts! In our previous post, we successfully developed a Playwright script that extracts crucial data from a website, including book titles, prices, and reviews. Today, we’ll build upon that foundation by adding a new feature—allowing our script to navigate through multiple pages by repeatedly clicking the "Next" button until no longer available.
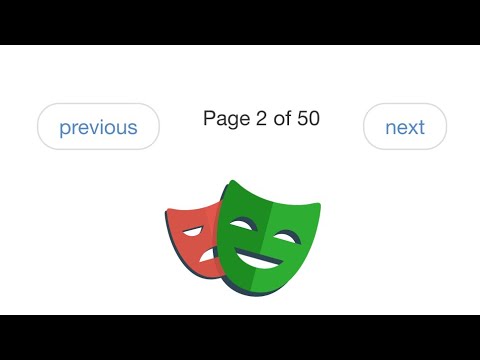
This blog was generated from a tutorial video you can watch here
Understanding the Next Button
To get started, we’ll need to identify the HTML element representing the “Next” button. Upon inspecting the webpage, we see that it’s not as straightforward as simply clicking on a single <a>
tag. The right selector is crucial to ensure our script functions correctly as we paginate through the data. So, after checking, we find the appropriate selector: pager next a
. This allows us to target the next page button directly.
Setting Up the Logic
Next, we need to integrate a control structure that lets our script click the next button. For this, a do while
loop would typically be our choice. This approach ensures that we scrape the first page regardless of whether any subsequent pages exist. However, we’ll now implement a more efficient solution using an asynchronous while
loop since it provides better control over the pagination flow.
Our Code Structure
Here’s a brief overview of our logic:
- Initialization: Start our while loop to continuously check for the visibility of the “Next” button.
- Scraping Data: If the button is visible, the script will click it and proceed to scrape the book data.
- Breaking the Loop: If the “Next” button becomes invisible, the loop breaks, and the script stops running.
while (true) {
const visible = await page.locator('pager next a').isVisible();
if (!visible) break; // Exit the loop if the button isn't visible
await page.locator('pager next a').click(); // Click the next button
// Code for scraping data goes here
}
Observations and Results
After implementing this looping logic, I ran the script to see how it performed. You may notice it would flash quite a bit as it rapidly navigates through pages, gathering data. However, the efficiency is remarkable—it quickly ripped through all available pages, and within moments, the browser would automatically close once there were no more pages to scrape.
By the end of the process, we had accumulated thousands of records much faster than before. The data was then exported as a CSV file, ready for analysis.
Conclusion
In today’s session, we explored how to automate the pagination of web scraping effectively. By checking for the visibility of the “Next” button and implementing a robust looping mechanism, we can extract large datasets with minimal manual intervention.
Stay tuned for our next episode where we’ll delve into additional testing techniques and strategies to optimize your automation scripts further. Thank you for following along, and happy sc