· tutorials · 3 min read
Mastering Puppeteer - Effective Strategies for Writing Automation Scripts
Welcome back to our ongoing series on writing automation scripts with Puppeteer! In this installment, we'll dive deeper into techniques for managing page loads and ensuring your scripts run smoothly without crashing.
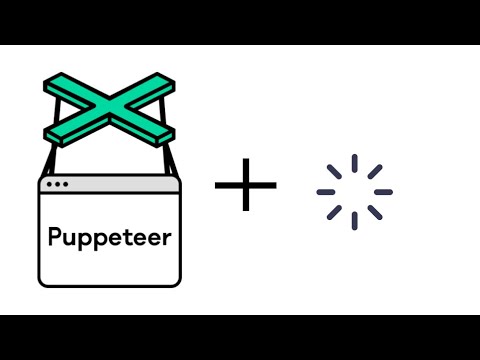
This blog was generated from a tutorial video you can watch here
Handling Non-Existent Elements
Have you ever encountered a situation where you attempt to click on a button in your Puppeteer script, only for it to crash because the element doesn’t exist? This is a common issue that stems from timing discrepancies between your script and the loading of the web page.
To effectively handle this, we need to implement a waiting mechanism. A straightforward method is to use waitForSelector
to ensure that the button or element is present before trying to click it. For instance, when waiting for a specific button, you can add the following code snippet to your main function:
try {
// Code that may throw an error
} catch (error) {
console.error(error);
await new Promise(resolve => setTimeout(resolve, 50000)); // Wait for debugging
}
This approach captures any potential errors and gives you extra time to identify the issue.
Waiting for Elements to Appear
Now, let’s say you have your selector confirmed. Instead of jumping directly to clicking, implement the waitForSelector
method. It allows you to specify that the process should only continue once the button is visible:
let selector = await page.waitForSelector('your-button-selector', { visible: true });
await selector.click();
This ensures that your script accurately waits for the element to become available, thus avoiding crashes.
Leveraging Network Requests
In scenarios where waitForSelector
may not suffice—such as dealing with dynamic loading or single-page applications—utilize Puppeteer’s network waiting options. There are four different waiting strategies you can implement:
- Load: Waits for the page to fully load.
- DOMContentLoaded: Waits until the initial HTML document has been completely loaded and parsed.
- Network Idle: This can be set to zero, meaning no network connections should be active for half a second.
- Network Idle 2: Allows up to two network connections for a brief moment.
For example, if you want to navigate to a new website and ensure it loads completely before proceeding, you might do something like this:
await page.goto('https://yourwebsite.com', { waitUntil: 'networkidle2' });
This will pause your script execution until the specified condition is met.
Handling Race Conditions
Race conditions can occur when you’re trying to click an element that also triggers a page navigation. To manage this situation, you can wrap your click action and the wait for navigation in a Promise.all()
, ensuring that both actions complete before moving forward:
await Promise.all([
page.waitForNavigation({ waitUntil: 'networkidle0' }),
page.click('your-button-selector'),
]);
This guarantees that your script successfully handles the navigation without missing clickable elements.
Conclusion
By employing these strategies, you can greatly enhance the reliability of your Puppeteer scripts. Handling errors, waiting for elements, and navigating within the context of network requests will save you from common pitfalls and improve script performance.
Stay tuned for more insights and techniques to streamline your automation process. Subscribe for updates, and happy scr